CSS Grid Basics
Demo starter files
It's not an overstatement to say that CSS Grid is completely changing the landscape of how we design on the web. In the next few lessons we'll look at what the CSS Grid Module can do, and how it is a tool built from the ground up to be responsive.
This relatively new feature in CSS landed simultaneously across nearly all of the major browsers in march 2017. It's one of the fastest and most synchronous rollouts of a new CSS feature ever, largely due to incredible popularity in the developer community. Web designers and developers flooded browser vendors with votes, feedback, and requests for the feature, helping to steer them toward making it a top priority.
What is Grid?
Like flexbox, Grid is a display mode that sets up a new formating context, and turns all of its direct children into grid items. The difference is that Grid allows layout in two dimensions - both columns and rows.
CSS Grid, as you can imagine, has a lot of properties that control its behavior. It even introduces a new unit, fr
, and new functions like repeat()
and minmax()
. Luckily though, Grid is still easy to get started with.
Setting up a Simple Grid
The first thing to do of course is to set display: grid
on the parent element that is to become our grid container.
.grid-container {
display: grid;
}
By itself this doesn't visually change anything until we tell the grid—at minimum—how many columns to make. We can define as many columns as we want, using any kind of CSS units. After that the grid algorithm handles the rest automatically, laying out each item across columns and rows.
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
}
Adding a few more properties for illustration, the code here creates a grid looks roughly like this:
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
Grid Gap
While you'll certainly still need margins and padding for things, you can easily add spacing between all of the grid items using the gap
property.
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
gap
creates spacing between the grid items.When CSS Grid was first introduced, the gap
property was called grid-gap
. If you see examples online referring to this property, assume they mean the same thing and just use gap
. This property has been given a more generalized name because it also works on flexbox now, too!
The fr Unit
It's great that we can mix and match units when defining a template for our grid, but ensuring our width settings add perfectly to 100% quickly becomes a lot of math. 5em + 64px + ??? = 100%? To solve this we have a new unit of measure: the fr
unit.
fr stands for fraction, and it basically means "whatever space is left". This allows us to use whatever width settings we want for the columns, and let the computer do the work of figuring out how much space is left. It even takes the gap
into account.
- 5em
- 1fr
- 64px
- 5em
- 1fr
- 64px
- 5em
- 1fr
- 64px
fr
helps take care of the math when defining grid templates.fr
also works a lot like flex-grow
and flex-shrink
ratios. You should always use integers when defining fr
units. If you don't need a specific (usually font based) width on any of your columns, you can simply set them up as a flexible ratio.
- 1fr
- 2fr
- 3fr
- 1fr
- 2fr
- 3fr
- 1fr
- 2fr
- 3fr
fr
can be used to create a ratio of sizes.Inspecting the Grid
"Cool, but there's already a lot happening here... how can I see what the heck is going on?"
Firefox, Chrome and Safari have excellent grid inspectors in their developer tools. Firefox was the first to develop this tool, but all of them work well now.
Open the inspector in the developer tools and select the element for any of the parent grid containers on this page. Look for a clickable flag next to the element in the DOM tree that says "grid". This will turn on an overlay that shows the grid. In Firefox, there is also a crosshatch icon next to the display: grid
property.
Once the overlay is turned on, switch from the "rules" tab over to the "layout" tab, and you can toggle checkboxes for other grid overlay settings, like line numbers and area names.
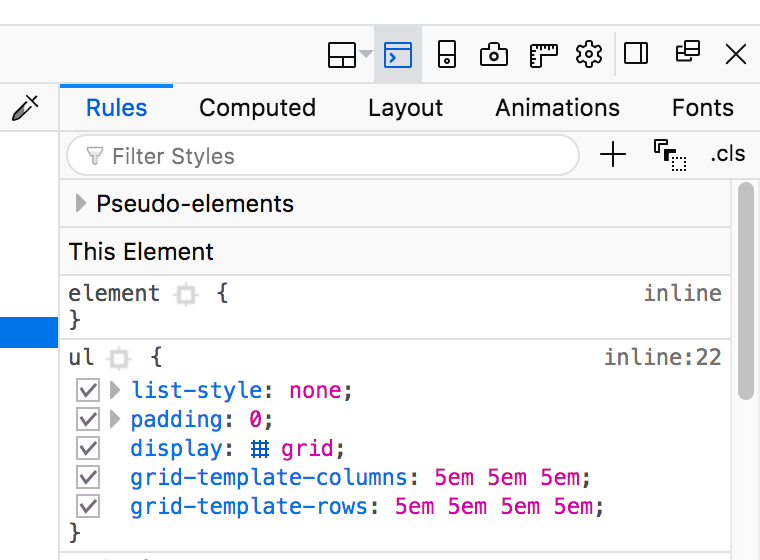
grid
setting.Let's switch over to the demo files and set up our first CSS Grid!